Overlay Sequence System
With the Overlay Sequence System, it is possible to display multiple input sequences on top of in-game objects. As shown in the interactive demo, each cube in the scene is overlaid with an overlay sequence.
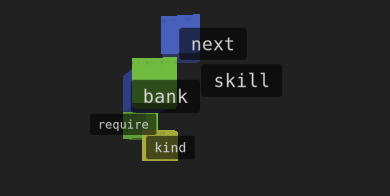
The Overlay Sequences System is composed of 3 components:
- The Overlay Sequence, which is a UI representation of an input sequence.
- The Overlay Sequence Manager, which manages the position and creation of overlay sequences within a canvas.
- The Overlay UI Settings, which control the behavior of overlay sequences.
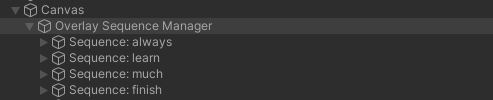
Quick Start
A ready-to-go canvas with the Overlay Sequence System can be found in the Typing Game Kit/Assets/Prefabs/
folder.
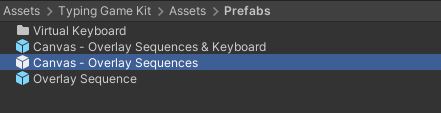
Simply drag and drop the Canvas - Overlay Sequences
prefab into the desired scene.
From this point, you can create new overlay sequences by calling the OverlaySequenceManager
component's CreateSequence
method.
InputSequence sequence = overlaySequenceManager.CreateSequence(text, target);
The CreateSequence
method will create a new overlay sequence with the provided text and target and return the corresponding InputSequence
.
Example Scene
The Typing Game Kit/Examples/Basics/Overlay Sequences
folder contains a scene that illustrates the usage of the overlay sequence system. In this scene, the OverlaySequencesExample
script will spawn several invisible objects in the scene and attach a sequence to each of them via the sequence overlay. Once a sequence has been typed another one will appear.
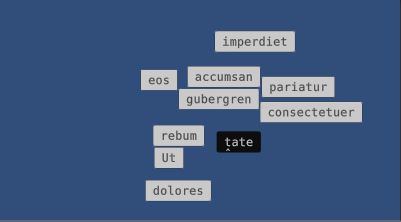
Additional Examples
Asteroid Typing 2D and Alien Typing 3D both use overlay sequences.
Components
Overlay Sequence
Each overlay sequence is represented by a UI object with the SequenceOverlay
component attached.
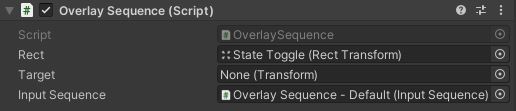
- The Rect specifies the
RectTransform
to use when avoiding overlap with other entities or remaining with its parent. - The Target defines the current in-game
Transform
that the overlay UI will overlay and follow. - The Input Sequence references the input sequence that the overlay sequence visualizes.
Several overlay sequence prefabs are already defined in the Typing Game Kit. The prefabs are composed of two InputSequenceUI
components that are toggled depending on whether their InputSequence
is targeted or not.
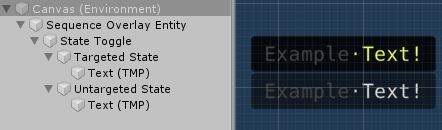
Overlay Sequence Manager
The OverlaySequenceManager
component has the following attributes in the inspector:
- The Settings define the
OverlaySettings
to use. - The Area reference a
RectTransform
that defines the allowed area of the overlay sequences. Only used if Restrict to Area setting is enabled. - The Camera reference defines what camera to use when projecting the sequences' position in the canvas. If undefined the scene's default camera will be used.
- The Sequence Prefab references the overlay sequence prefab used for new input sequences.
Screen Space - Overlay
The SequenceOverlayManager
needs to be inside a Canvas with the Screen Space - Overlay rendering mode to function properly.
Overlay UI Settings
The OverlayUISettings
component defines the behavior of the overlay sequences. It has the following attributes:
- Restrict to Area - If toggled overlay sequences will be restricted to the sequence overlay's area
RectTransform
. - Movement Smoothness - Defines how smooth the movement of the sequences will be. Set to zero to disable.
- Avoidance Strength - Determines how much the sequences will be repelled from each other.
- Use Distance Scaling - Enables/disables the scaling of entities depending on their target's distance from the camera.
Distance Scaling Details
If the Distance Scaling option is enabled then overlay sequences size will be scaled based on their distance to the camera according to the following scheme:
- If closer than Closest Distance the overlay UI will have the Closest Distance Scale.
- If further away than the Furthest Distance the overlay UI will have the Furthest Distance Scale.
- If in between the furthest and closest distances the scale will be linearly interpolated between the two scales.