Input Sequences
At the core of the Typing Game Kit is the InputSequence
component. In essence, it is a MonoBehaviour
that represents a typeable text sequence.

Before an input sequence may be typed, it has to become targeted. A sequence may automatically become targeted once its required input is typed.
A targeted sequence will accept or reject all further keyboard input until it has been completed or manually untargeted.
Inspector Attributes
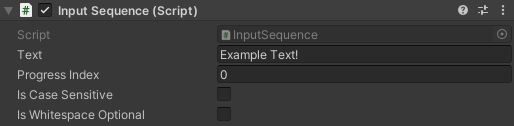
- Text - Text string that defines the required input of the input sequence.
- Progress Index - The index of the currently required input of the input sequence text.
- Is Case Sensitive - If toggled, the letter casing of typed input must match to be accepted by the input sequence.
- Is Whitespace Optional - If enabled, whitespace will be optional and the next non-whitespace character would also be an acceptable input.
InputSequence Events
The overarching pattern in using an InputSequence
is to subscribe to its events and thereby invoke desired gameplay interactions.
For instance, in the Asteroid Typing 2D example, the events of an input sequence are subscribed to like this:
sequence.OnInputAccepted += delegate { _player.FireAt(asteroid); };
sequence.OnCompleted += delegate { asteroid.Explode(); };
In the code above, we define an event handler that will fire on the asteroid when input is accepted
. Similarly, we add an event handler to OnCompleted
to destroy the asteroid once the sequence has been completed. In both cases, the event handlers are some game logic wrapped in anonymous methods using the delegate
syntax.
Have a look at the scripting interface section for a listing of all available events.
InputSequenceManager events
The InputSequenceManager
, accessible through InputSequence.Manager
, has several events that are raised when something happens to any active sequence.
For instance, we can do the following if we want to play an error sound when input is rejected by any sequence:
private void Start()
{
InputSequence.Manager.OnInputRejected += PlayErrorSound;
}
private void PlayErrorSound()
{
// Play the errors sound in here
}
It is also a good idea of unsubscribing an event handler before it goes out of scope in the OnDestroy
method:
private void OnDestroy()
{
InputSequence.Manager.OnInputRejected -= PlayErrorSound;
}
Have a look at the scripting interface section for all available events for the InputSequenceManager
.
Input Sequence UI
The InputSequenceUI
component is the default way of displaying the state of the input sequence inside a UI canvas. The InputSequenceUI
depends on TextMesh Pro and its text formatting features.
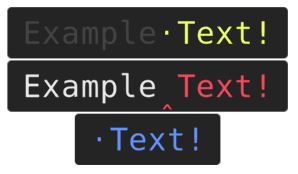
Inspector Attributes
The InputSequenceUI
has several attributes that define how the input sequence will be displayed.
- Sequence - A reference to the input sequence being displayed.
- Text - A Text Mesh Pro component that displays the text output.
- Completed Color - Color of the completed portion of the text.
- Remaining Color - Color of the remaining text
- Error Color - Color of required input if rejected input has been received.
- Hide Completed Text - If enabled the completed portion of the text will be hidden.
- Position Indicator - The specified symbol will be drawn to indicate the next position in the sequence.
- Space Replacement - Specifies the symbol to use to replace the space character when its input is required.
Adjusting the position indicator
By default, the position indicator is represented by the non-spacing character U+032D. The indicator's position can be adjusted in Text Mesh Pro's SDF font asset's Glyph Table section in the inspector.
Example Scene
The Input Sequence example scene combines all the features in this section and can be found within the Typing Game Kit/Examples/Basics/Input Sequence
folder. The scene contains an InputSequence
, InputSequenceUI
, and a TextChanger
script that changes the input sequence's text once it has been completed.
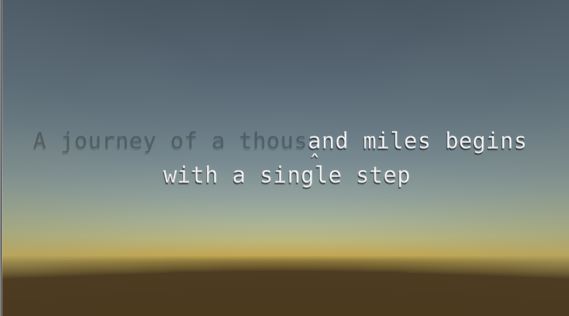